[Javascript] 노마드코더바닐라 JS로 크롬 앱 만들기 3
노마드 코더 Nomad Coders
코딩은 진짜를 만들어보는거야!. 실제 구현되어 있는 서비스를 한땀 한땀 따라 만들면서 코딩을 배우세요!
nomadcoders.co
노 마트 코더 Vanilla JS는 크게 3개의 수업으로 나눠져 있는데, 이번에는 마지막 수업에 관한 노트
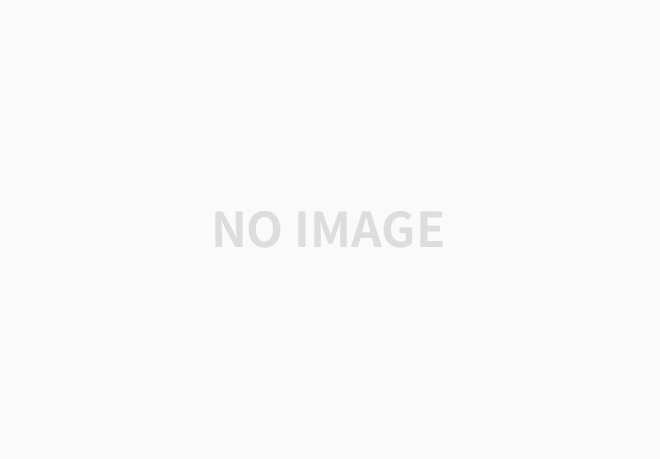
3.0 JS Clock
/* this is for js file */
const clockContainer = document.querySelector(".js-clock"),
clockTitle = clockContainer.querySelector("h1")
function getTime(){
const date = new Date();
const minutes = date.getMinutes();
const hours = date.getHours();
const seconds = date.getSeconds();
clockTitle.innerText=`${hours}:${minutes}:${seconds}`
}
function init(){
getTime();
}
init()
/* html file */
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8" />
<meta name="viewport" content="width=device-width" />
<title>NomadCoder JS</title>
<link href="style.css" rel="stylesheet" type="text/css" />
</head>
<body>
<div class="js-clock">
<h1 class="js-title">0:00</h1>
</div>
<script src="clock.js"></script>
</body>
</html>
3 real time clock
setInterval function
setInterval(functionname, second)
/* for examaple */
function sayHi(){
conssole.log("hi);
}
setInterval(sayHi, 1000)
// every one second sayhi
which mean you can apply this in the js for realtime clock
function init(){
getTime();
setInterval(getTime, 1000)
}
additionally
ternary operator ("mini if")
clockTitle.innerText=`${hours}:${minutes}:${seconds < 10 ? `0${seconds}`: seconds}`;
if the second is less than 10 => add 0
if the second is biger than 10 => just show the second
const clockContainer = document.querySelector(".js-clock"),
clockTitle = clockContainer.querySelector("h1")
function getTime(){
const date = new Date();
const minutes = date.getMinutes();
const hours = date.getHours();
const seconds = date.getSeconds();
clockTitle.innerText=`${hours < 10 ? `0${hours}` : hours}:${minutes < 10 ?`0${minutes}`: minutes}:${seconds < 10 ? `0${seconds}`: seconds}`;
}
function init(){
getTime();
setInterval(getTime, 1000)
}
init()
3.2 Saving user name
local storage
save small piece of js information in the browser?
querySelector: gets any selectors like class, tag, id --> gets the first one it finds
querySelectorAll: gets all of them;
getElementById
- get elements by class names, which will give you an array of all the elements it finds
getElementByTagName: gets elements by tag names (literally)
3.3 saving user name
const formcontainer = document.querySelector(".js-form"),
input= formcontainer .querySelector("input"),
greeting = document.querySelector(".js-greeting");
/* getting access to the selected object in html */
const USER_LS = "currentUser"; //local storage
SHOWING_CN= "showing"; // css -> showing
// handleSubmit even => cancelthe default event -> store the name ->
// save the name -> greeting
function handleSubmit(){
event.preventDefault();
const currentValue = input.value;
console.log(currentValue);
paintGreeting(currentValue);
saveName(currentValue);
}
// save name in the local storage
function saveName(text){
localStorage.setItem(USER_LS, text);
}
// ask the name first show the form to ask and get the event handled
function askName(){
formcontainer.classList.add(SHOWING_CN);
formcontainer.addEventListener("submit", handleSubmit)
}
// greeting msg with name
function paintGreeting(text){
formcontainer.classList.remove(SHOWING_CN);
greeting.classList.add(SHOWING_CN);
greeting.innerText = `What's up ${text}!`
}
function loadName(){
const currentUser = localStorage.getItem(USER_LS);
if(currentUser === null){
askName();
}else {
paintGreeting(currentUser);
}
}
function init(){
loadName();
}
init();
3.4 To do part 1
appendChild(): puts something inside of its father
3.5 To do part2
JSON : JavaScript Object Notation
take js obejct to string or vice versa
for each => function for array
there are function for string and object too
MDN foreach explantion
developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/forEach
Array.prototype.forEach() - JavaScript | MDN
The forEach() method executes a provided function once for each array element. The source for this interactive example is stored in a GitHub repository. If you'd like to contribute to the interactive examples project, please clone https://github.com/mdn/in
developer.mozilla.org
3.6 To do part3
1. deleting html
2. deltting from the storage
console.dir(event.target.parentNode);
filter = runs a fuinction like foreach fu
nction for each item
make new array by filtering?
const variable cant change
const toDos = [] = > chagne to let toDos = [];
const toDoform = document.querySelector(".js-toDoForm"),
toDoInput = toDoform.querySelector("input"),
toDoList = document.querySelector(".js-toDoList");
// access to the html object
const TODOS_LS = "toDos"; // local storage
let toDos =[]; // emptry array for the todo list
function saveTodos(){
localStorage.setItem(TODOS_LS, JSON.stringify(toDos));// JSON.stringify to make it as string
// local sotrage can only save string values.
// js object --> string
}
function paintToDo(text){
const potato =document.createElement("li"); // create li tag
const delBtn = document.createElement("button"); // create button
delBtn.innerHTML=" ❌ "; // button inside
delBtn.addEventListener("click",deleteToDo); // button event if click -> delte todo
const onion = document.createElement("span");
const newId= toDos.length+1; // li id
onion.innerText =text; // text that you write in the todo
potato.appendChild(onion);
potato.appendChild(delBtn);
toDoList.appendChild(potato);
potato.id =newId; // give id to li
const toDoObj = {
text:text,
id: newId
}; // this object to the toDos array
toDos.push(toDoObj); // add the array object
saveTodos();
}
function loadToDos(){
const loadedToDos = localStorage.getItem(TODOS_LS);
if (loadedToDos !== null){
console.log(loadedToDos);
const parsedToDos = JSON.parse(loadedToDos); // take string back to object
console.log(parsedToDos);
parsedToDos.forEach(function(eachItem){
paintToDo(eachItem.text);
})// each task it will shows
// eachItem .text or id or both?
}
}
function handleSubmit(event){
event.preventDefault();
const currentValue= toDoInput.value;
paintToDo(currentValue);
toDoInput.value="";
}
function deleteToDo(event){
//console.log(event.target.parentNode);
const btn = event.target;
const li = btn.parentNode;
toDoList.removeChild(li);
const cleanToDos= toDos.filter(function (toDo)
{
return toDo.id !== parseInt(li.id);
});// check if li id is exist of
toDos = cleanToDos;
saveTodos();
}
function init(){
loadToDos();
toDoform.addEventListener("submit", handleSubmit)
}
init()
3.8 background img
무료 색 찾는 사이트?
generating number : math.random() * 5 = generate randome number 1 - 5
math.floor(mth.random()*5) to get rid of the float number
1. first generate randomnumber
2. connect with img number
3. appenmd to body
with bit of css styling + background.js
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8" />
<meta name="viewport" content="width=device-width" />
<title>NomadCoder JS</title>
<link href="style.css" rel="stylesheet" type="text/css" />
<link rel="preconnect" href="https://fonts.gstatic.com" />
<link
href="https://fonts.googleapis.com/css2?family=Heebo:wght@100;200;300;400&display=swap"
rel="stylesheet"
/>
</head>
<body>
<div class="js-clock">
<h1 class="js-title">0:00</h1>
</div>
<form class="js-form form">
<input type="text" placeholder="What is your name?" />
</form>
<h4 class="js-greeting greeting"></h4>
<form class="js-toDoForm toDoForm">
<input type="text" placeholder="Write a to Do" />
</form>
<ul class="js-toDoList">
<!-- <li id="1"></li> -->
</ul>
<script src="clock.js"></script>
<script src="greeting.js"></script>
<script src="todo.js"></script>
<script src="background.js"></script>
</body>
</html>
* {
font-family: "Heebo", sans-serif;
}
body {
background-color: #3498db;
text-align: center;
font-size: large;
border: 3px solid;
border-radius: 20px;
}
.btn {
cursor: pointer;
}
.clickedColor {
color: red;
}
.form,
.greeting {
display: none;
}
.showing {
display: block;
}
ul {
list-style: none;
text-emphasis: bold;
}
@keyframes fadeIn {
from {
opacity: 0;
}
to {
opacity: 1;
}
}
.bgImage {
position: abolute;
top: 0;
left: 0;
width: 100%;
height: 100%;
z-index: -1;
animation: fadeIn 0.5s linear;
}
const body = document.querySelector("body");
const IMG_NUMBER = 13;
/*
function handleImgLoad(){
console.log("finished load");
}
*/
function paintImg(imgNumber){
const image = new Image();
image.src =`img/${imgNumber}.jpg`;
body.appendChild(image);
// image.addEventListener("loadend" , handleImgLoad) we dont need this cuz
// we are not downloading any img via API
image.classList("bgImage");
}
function genRandom(){
const number = Math.floor(Math.random() * IMG_NUMBER);
return number;
}
function init(){
const randomeNumber = genRandom();
paintImg(randomeNumber);
}
init();
3.8 weather
1. get my location -> save it in local storage
2. weather API key download ;
3.9
API = Application Programming Interface
simple way to download data from other server
only date get it from other server only data not the desing
how the machines communicate eachother
1. using API to get weather information
2. put the weather in the html (show the weatehr)
const weatehr = document.querySelector(".js-weather");
const COORDS ='coords';
const API_KEY = "dc011e1ff1445e517f4e48628aebac04"; // api key for the weather
function getWeather(lat, lng){
fetch(`https://api.openweathermap.org/data/2.5/weather?lat=${lat}&lon=${lng}&appid=${API_KEY}&units=metric`
).then(function(response){ //network response
return(response.json())}) // you get the JSON response
.then(function(json){
console.log(json);
const temperature = json.main.temp;
const place=json.name;
weatehr.innerText = `${temperature} C in outside (@${place})`
});
}
//then => after one action you do next action
function saveCoords(coordsObj){
localStorage.setItem(COORDS, JSON.stringify(coordsObj));
}
function handleGeoSucess(position){
console.log(position);
const latitude =position.coords.latitude;
const longitude =position.coords.longitude;
const coordsObj = {
latitude,
longitude
};// latitude =latitude => latitude,
saveCoords(coordsObj);
getWeather(latitude, longitude);
}
function handleGeoError(position){
console.log("can not access your location!");
}
function askForCoords(){
navigator.geolocation.getCurrentPosition(handleGeoSucess, handleGeoError);
// api navigator
}
function loadCoords(){
const loadedCoords = localStorage.getItem(COORDS);
if(loadedCoords === null){
askForCoords();
}else{
const parseCoords = JSON.parse(loadedCoords);
console.log(parseCoords);
getWeather(parseCoords.latitude, parseCoords.longitude);
}
}
function init(){
loadCoords();
}
init();
Vanilla JS - Finished
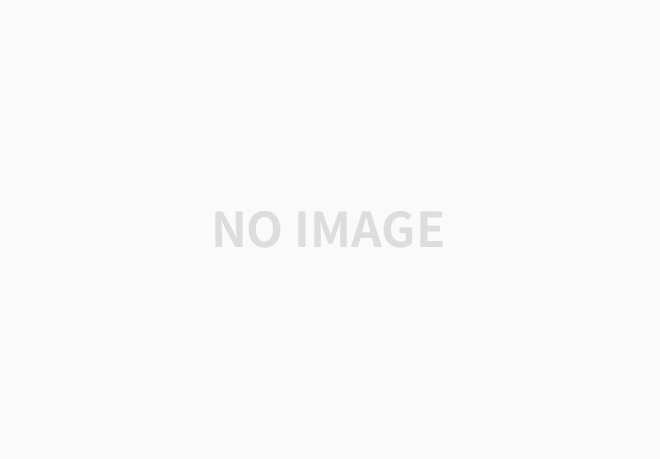
강의를 다들으면 저렇게 다한 강의가 나오는것같은데 무료 강의도 괜찮은게 많은것같아서
이번 방학동안 노마드코더 무료강의들을 다뿌시고 챌린지 프로그램을 끝내면 쿠폰도 준다는것같은데
쿠폰 받아서 본강의도 들을수있으면좋을것같다.
다음주 쯤에 챌린지? 프로그램이 있다고들은것같은데
챌린지 프로그램을하면서 다같이 코딩을 하고 수업외적으로 숙제같은것들이있는것같음
'IT > Web Programming' 카테고리의 다른 글
[NomadeCoder] HCSS Lay out 2주 완성반 challenge 1/14day (0) | 2021.01.12 |
---|---|
[Nomadecoder] Vanilla JS Challenge Day 1 /14 (0) | 2021.01.11 |
[스파르타코딩] 크리스마스 코딩 및 스파르타코딩 후기 (0) | 2021.01.02 |
[Javascript] 노마드코더바닐라 JS로 크롬 앱 만들기 2 (0) | 2020.12.31 |
[Javascript] 노마드코더바닐라 JS로 크롬 앱 만들기 1 (0) | 2020.12.29 |